Step Into Gerber Generation
Author
Tony Wang
Date Published
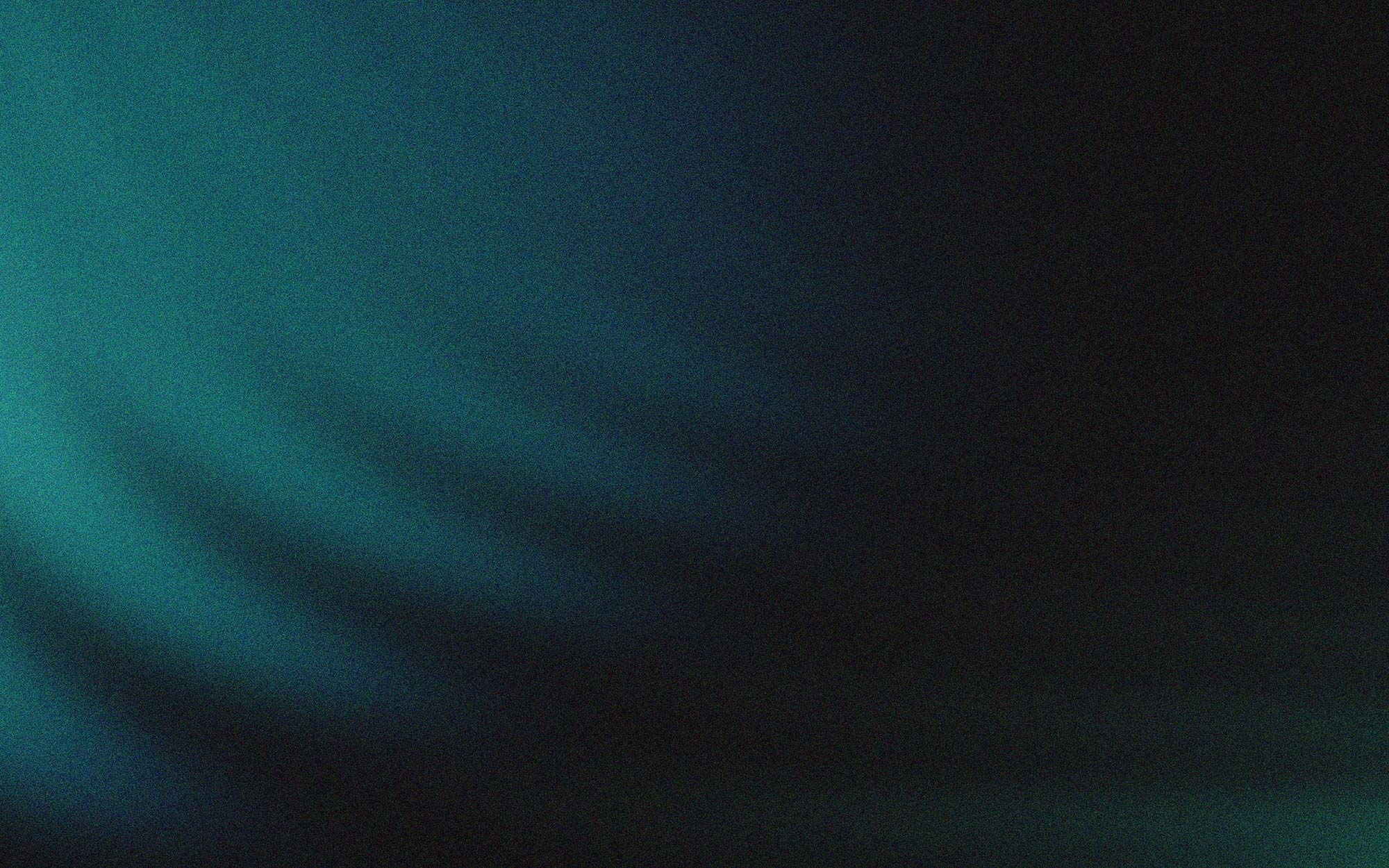
Understanding the Gerber format is essential. Gerber RS-274X uses a series of commands like D01 for draws, D02 for moves, and D03 for flashes. Aperture definitions are in the header. So, for a Python class, I need to generate these commands based on the component's dimensions.
The user wants a Python class that takes dimensions and layer, then outputs Gerber code. Let's outline the steps:
1. Aperture Management: Each shape (pad, line) needs an aperture. The class should track apertures and reuse them to minimize the list.
2. Coordinate System: Gerber uses units in mm or inches, with precision. The class needs to handle coordinates with the correct precision.
3. Layer Handling: Different layers (copper, mask, silkscreen) have specific rules. The class should map inputs to the correct Gerber layer commands.
4. Element Conversion: Convert component dimensions into Gerber primitives. For example, a rectangular pad would be a rectangle aperture flashed at a position.
Potential challenges include handling different shapes (circles, rectangles, polygons), managing aperture macros if needed, and ensuring correct Gerber syntax. Also, thermal reliefs or complex polygons might be more complicated, but the user might start with basic shapes.
Testing with a simple example would help. For instance, a rectangular SMD pad on the top layer. The Python class would define the rectangle aperture, then flash it at the given coordinates. The Gerber output should include the aperture definition and the flash command.
I should also consider units and scaling. KiCad uses millimeters by default, so the Python class should accept dimensions in mm and convert them to Gerber units (e.g., 1mm = 100000 units with 3 decimal places).
Now, putting this into a Python class structure. The class needs methods to add pads, lines, etc., manage apertures, and generate the Gerber code. The user would input dimensions, and the class would translate that into the appropriate Gerber elements.
For example, if the user inputs a pad with width 2mm, height 1mm, position (10,20), the class would check if a 2x1mm rectangle aperture exists, define it if not, then generate a D03 command at X1000000Y2000000 (assuming 3 decimal places).
I should also mention limitations. Without the full context of the PCB (like other components, design rules), this class would generate isolated Gerber snippets. Integration with KiCad's DRC might be needed for manufacturability, but that's beyond the scope here.
Finally, provide a code outline and example usage. The code would include aperture tracking, command generation, and layer assignment. The example would show creating a footprint with pads and lines, then outputting the Gerber code.
1class GerberFootprintGenerator:2 def __init__(self, layer="F.Cu", units="mm", precision=6):3 self.layer = layer # Target layer (e.g., "F.Cu", "F.SilkS")4 self.units = units5 self.precision = precision # Gerber decimal places (e.g., 6 = 1 micron)6 self.apertures = {} # {"shape_params": aperture_id}7 self.aperture_id = 10 # Start aperture IDs from 108 self.commands = [] # Gerber commands buffer9 self._header()1011 def _header(self):12 # Initialize Gerber header13 self.commands.append("%TF.FileFunction,{}*%".format(self.layer))14 self.commands.append("%FSLAX{0}{0}Y{0}{0}*%".format(self.precision))15 self.commands.append("%MO{}*%".format(self.units.upper()))1617 def _add_aperture(self, shape, params):18 # Define aperture if not already exists19 key = f"{shape}{params}"20 if key not in self.apertures:21 self.apertures[key] = self.aperture_id22 self.commands.append("%ADD{} {},{}*%".format(23 self.aperture_id, shape, ",".join(map(str, params))24 ))25 self.aperture_id += 126 return self.apertures[key]2728 def add_rect_pad(self, x, y, width, height):29 # Add rectangular pad (e.g., SMD pad)30 aperture_id = self._add_aperture("R", [width, height])31 cmd = "X{}Y{}D03*".format(32 int(x * 10**self.precision),33 int(y * 10**self.precision)34 )35 self.commands.append(cmd)3637 def add_circ_pad(self, x, y, diameter):38 # Add circular pad (through-hole)39 aperture_id = self._add_aperture("C", [diameter])40 cmd = "X{}Y{}D03*".format(41 int(x * 10**self.precision),42 int(y * 10**self.precision)43 )44 self.commands.append(cmd)4546 def add_outline(self, points, line_width=0.15):47 # Add silkscreen outline (polygon)48 aperture_id = self._add_aperture("C", [line_width])49 self.commands.append("G36*") # Start polygon50 x_prev, y_prev = None, None51 for x, y in points:52 if x_prev is not None:53 # Draw line from previous point54 self.commands.append("X{}Y{}D02*".format(55 int(x_prev * 10**self.precision),56 int(y_prev * 10**self.precision)57 ))58 self.commands.append("X{}Y{}D01*".format(59 int(x * 10**self.precision),60 int(y * 10**self.precision)61 ))62 x_prev, y_prev = x, y63 self.commands.append("G37*") # End polygon6465 def generate_gerber(self):66 # Finalize Gerber code67 self.commands.append("M02*")68 return "\n".join(self.commands)
Generate Gerber code for a 2x3mm rectangular pad and a silkscreen outline:
1# Initialize generator for top copper layer2gen = GerberFootprintGenerator(layer="F.Cu")34# Add a rectangular pad at (10mm, 20mm)5gen.add_rect_pad(x=10, y=20, width=2, height=3)67# Add a silkscreen outline (rectangle)8outline_points = [9 (9, 19),10 (11, 19),11 (11, 21),12 (9, 21),13 (9, 19)14]15gen.add_outline(outline_points, line_width=0.15)1617# Generate Gerber code18gerber_code = gen.generate_gerber()19print(gerber_code)
Output Gerber Snippet:
1%TF.FileFunction,F.Cu*%2%FSLAX66Y66*%3%MOMM*%4%ADD10R,2,3*%5X10000000Y20000000D03*6%ADD11C,0.15*%7G36*8X9000000Y19000000D02*9X11000000Y19000000D01*10X11000000Y21000000D01*11X9000000Y21000000D01*12X9000000Y19000000D01*13G37*14M02*
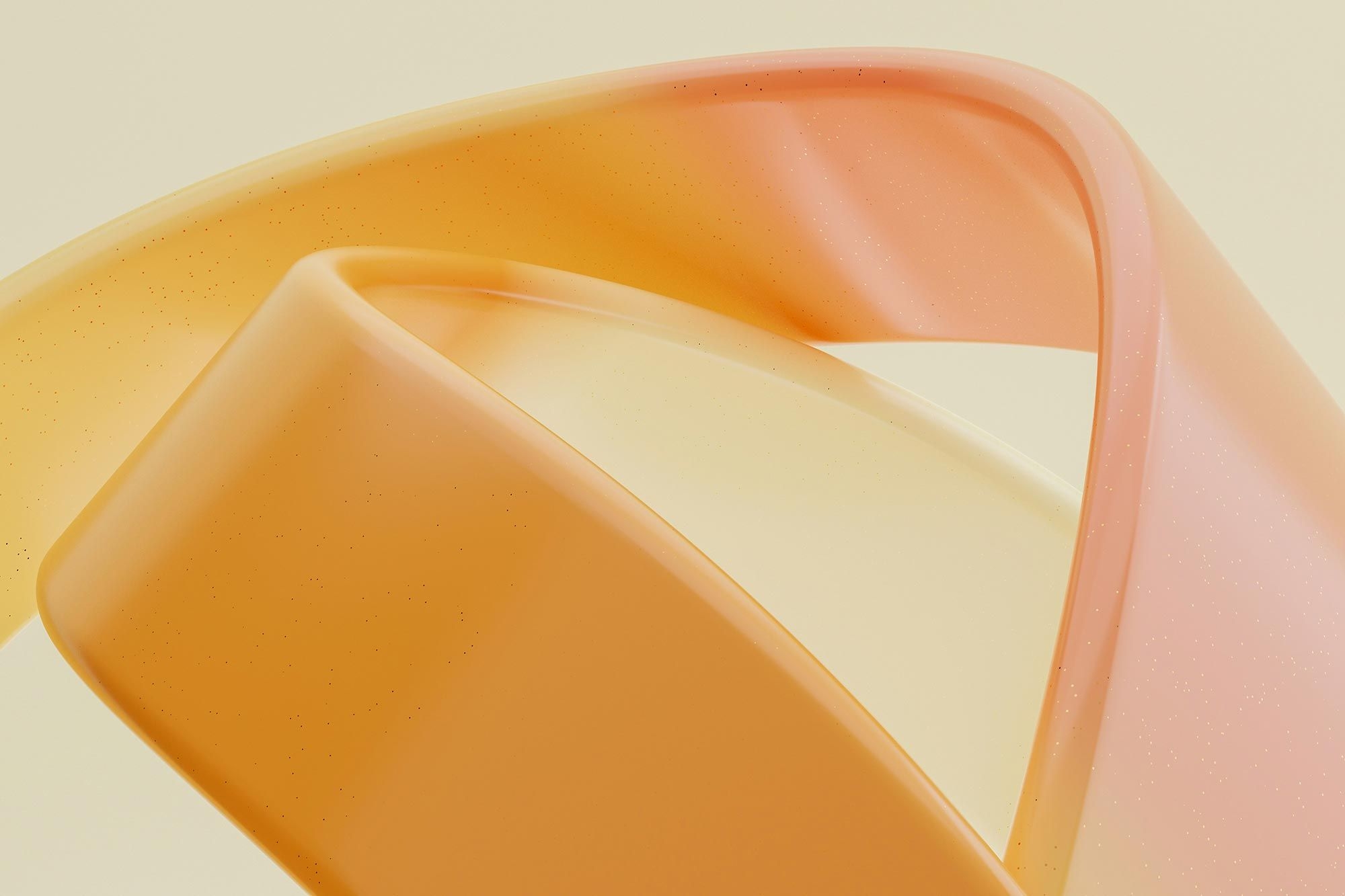